reactの環境設定がまだな方はこちらからお願いします。
https://kazunaka.com/react-helloworld/
プロジェクトの作成
コマンドでcreate-react-appを実行して、プロジェクトを作成していきます。
$ create-react-app count_app
「Success!」が表示されたらプロジェクトができていると思いますので、
作成したプロジェクトへ移動します。
$ cd !$
カウントアプリの実装
src/App.jsを編集していきます。
import React, { Component } from 'react';
// カウント値
var countValue:number = 1;
const App = () => {
return <Counter />
}
class Counter extends Component
{
constructor(props)
{
super(props);
this.state = {
value : 0
}
}
// インクリメント
increment = () => {
this.setState({
value : this.state.value + countValue
});
}
// デクリメント
decrement = () => {
if (0 <= this.state.value - countValue) {
this.setState({
value : this.state.value - countValue
});
}
}
render()
{
return (
<div>
<div>
カウント値 : {this.state.value}
</div>
<div>
<button type="button" onClick={this.increment}> +{countValue}ボタン </button>
<button type="button" onClick={this.decrement}> -{countValue}ボタン </button>
</div>
</div>
);
}
}
export default App;
カウントアプリの実行
アプリの実行コマンドを入力します。
$ yarn start
以下のように画面になりましたでしょうか。
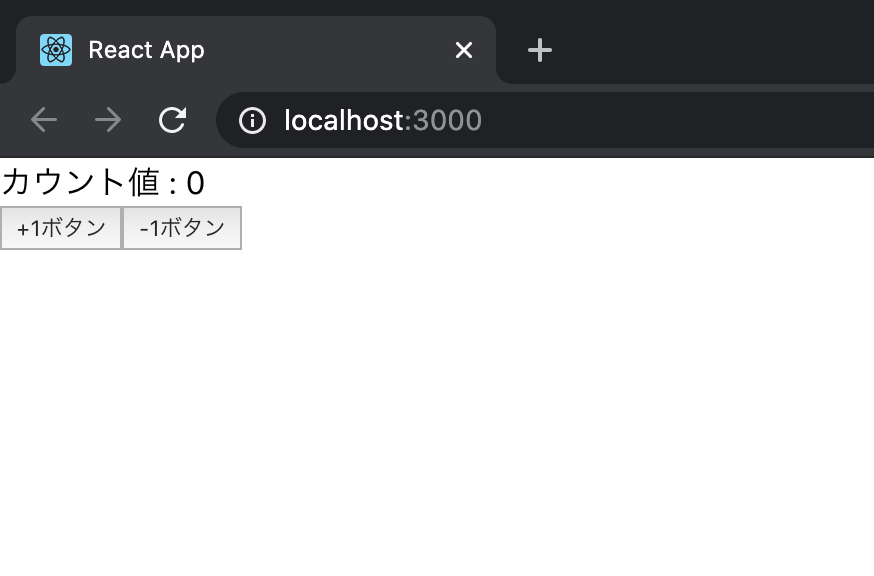
ボタンを押してみて正常にカウント値が増減したら成功です。
(エラー起きた場合は、コードの間違え探しです、、。)
カウント値はマイナスにならないよう制御をかけています。
*ちなみに、、、
*小数点だと丸め誤差というのが起きてしまい変な数値になると思います。
*2進数での計算を内部でしているのでうまく10進数にできないのです。
*気になる方は別で「丸め誤差」を調べてみてください。
変動数を変えたい場合は
// カウント値
var countValue:number = 1;
の値を変更してみてください。
以上、reactでカウントアプリを作ってみようでした!
コメント