reactの環境設定がまだな方はこちらからお願いします。
https://kazunaka.com/react-helloworld/
前準備
今回「redux, react-redux」を使うので、インストール未の方は以下を実行してください。
分からない方はとりあえずinstallを実行してもらって問題ないと思います。
$ npm install --save redux
$ npm install --save react-redux
プロジェクトの作成
コマンドでディレクトリを移動して、プロジェクトを作成しましょう。
$ create-react-app redux_count_app
「Success!」が表示されたらプロジェクトができていると思いますので、
作成したプロジェクトへ移動します。
$ cd !$
パッケージを使用するためのコマンドをたたきます。
$ yarn add redux
$ yarn add react-redux
ファイル構成の作成
以下のようなファイル構成になります。
src
├── actions
└── index.js
├── components
└── Counter.js
├── constants
└── countConst.js
├── reducers
├── index.js
└── count.js
├── index.js
└── serviceWorker.js
このファイル構成をコマンドで作成していきましょう。
$ cd src
$ mkdir actions components reducers constants
$ touch actions/index.js
$ touch components/Counter.js
$ touch constants/countConst.js
$ touch reducers/index.js
$ touch reducers/count.js
$ cd ../
ファイルを編集
ディレクトリ「src」以下のファイルを編集していきます。
actions/index.jsを編集していきます。
カウントのアクションを書いていきます。
export const INCREMENT = "INCREMENT";
export const DECREMENT = "DECREMENT";
export const increment = () => ({
type : INCREMENT
});
export const decrement = () => ({
type : DECREMENT
});
次にconstants/countConst.jsを編集して
カウントをしていく値を決めます。
// カウント値
export const COUNT_VALUE:number = 1;
reducers/count.jsを編集します。
stateの情報を更新します。
import { COUNT_VALUE } from '../constants/countConst';
import {
INCREMENT,
DECREMENT,
} from '../actions';
const initialize = { value : 0 };
export default (count = initialize, action) => {
switch (action.type) {
case INCREMENT:
return { value : count.value + COUNT_VALUE };
case DECREMENT:
if (0 <= count.value - 1 ) {
return { value : count.value - COUNT_VALUE };
}
return count
default:
return count
}
}
次はreducers/index.jsを編集します。
import { combineReducers } from 'redux';
import count from './count';
export default combineReducers({ count });
次にindex.jsを編集します。
Reduxをcomponentに対して利用可能にするために
ProviderでCounterをラップします。
import React from 'react';
import ReactDOM from 'react-dom';
import Counter from './components/Counter';
import { Provider } from 'react-redux';
import { createStore } from 'redux';
import reducer from './reducers';
import * as serviceWorker from './serviceWorker';
const store = createStore(reducer);
ReactDOM.render(
<Provider store={store}>
<Counter />
</Provider>,
document.getElementById('root')
);
// If you want your app to work offline and load faster, you can change
// unregister() to register() below. Note this comes with some pitfalls.
// Learn more about service workers: https://bit.ly/CRA-PWA
serviceWorker.unregister();
次はcomponents/Counter.jsを編集します。
こちらが実際に表示される画面側になります。
import React, { Component } from 'react';
import { connect } from 'react-redux';
import { increment, decrement } from '../actions';
import { COUNT_VALUE } from '../constants/countConst';
class Counter extends Component {
render() {
return (
<React.Fragment>
<div>
カウント値 : {this.props.value}
</div>
<div>
<button onClick={this.props.increment}> +{COUNT_VALUE}ボタン </button>
<button onClick={this.props.decrement}> -{COUNT_VALUE}ボタン </button>
</div>
</React.Fragment>
);
}
}
const mapStateToProps = state => ({ value : state.count.value });
const mapDispatchToProps = ({ increment, decrement });
export default connect(mapStateToProps, mapDispatchToProps)(Counter);
アプリを実行
コマンドでアプリを実行しましょう。
$ yarn start
画面がうまく表示されましたでしょうか。
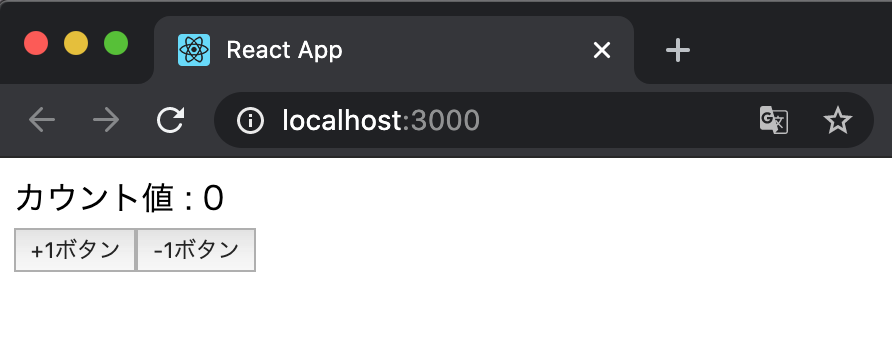
ボタンを押してみて正常にカウント値が増減したら成功です。
(エラー起きた場合は、コードの間違え探しをしましょう。)
以上、React + Reduxでカウントアプリの作成でした。
コメント